Creating a Telegram bot to interact with
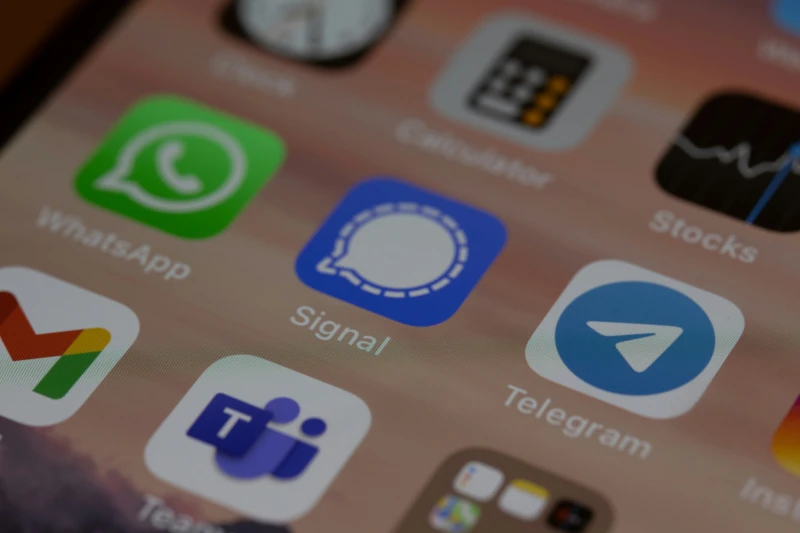
The steps are as follows:
Start chat with BotFather on desktop Telegram
create a new bot using
/newbot
give the bot a name eg. Steven's Demo Bot
give the bot a username eg. StevensDemoBot
take note of the Telegram token
start a conversation with Steven's Demo Bot
hit the Telegram API
getUpdates
endpoint with your token eghttps://api.telegram.org/bot000000000:AAFjSq4AMkx1JkQS4woTlyKlXeftv6mgu-I/getUpdates
if there's no update, add a message to the conversation again
visit the API again and take a note of the chat id
send an http request to
https://api.telegram.org/bot000000000:AAFjSq4AMkx1JkQS4woTlyKlXeftv6mgu-I/sendMessage?chat_id=000000000&text=Feed%20Me
to send a message
Mainly I'm doing this in the context of a PHP Laravel application but it could totally be language agnostic.
Here's the simple Telegram service I use
<?php
namespace App\Services;
use Illuminate\Support\Facades\Http;
use Illuminate\Support\Facades\Log;
class TelegramService
{
public function __construct(
private string $botToken = '',
private string $chatId = ''
) {
$this->botToken = $this->botToken ?: config('services.telegram.token');
$this->chatId = $this->chatId ?: config('services.telegram.chat_id');
}
public function sendMessage($message): bool
{
$response = Http::post("https://api.telegram.org/bot{$this->botToken}/sendMessage", [
'chat_id' => $this->chatId,
'text' => $message,
]);
if ($response->successful()) {
Log::info("Telegram message sent successfully");
return true;
} else {
Log::error("Failed to send Telegram message: " . $response->body());
return false;
}
}
}